- jQuery is a lightweight, "Write Less, Do More", JavaScript library.
- The purpose of jQuery is to make it much easier to use JavaScript on your website.
- jQuery takes a lot of common tasks that requires many lines of JavaScript code to accomplish, and wraps it into methods that you can call with a single line of code.
- jQuery also simplifies a lot of the complicated things from JavaScript, like AJAX calls and DOM manipulation.
- Document Object Model
- is the Backbone of every webpage
- web browser parses HTML and Javascript and maintains
a Data Model of what should be displayed to the user
The jQuery Library Contains the Following Features:
- HTML/DOM manipulation
- CSS manipulation
- HTML event methods
- Effects and animations
- AJAX
- Utilities
- jQuery has plugins for almost any task out there.
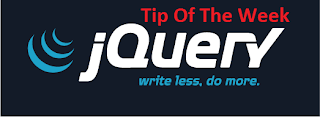
What is a jQuery Selector?
Selector Syntax
- A Selector identifies an HTML element tag that is used to manipulate with jQuery code.
- Selectors allow page elements to be selected
Selector Syntax
$(Selector Expression) OR jQuery(Selector Expression)
What are the Types of jQuery Selectors?
1. Class Selectors
$(".intro") - All elements with class="intro"
2. ID Selectors
$("#lastname") - The element with id=lastname
3. Element Selectors
$("p") - All p elements
How to Caching jQuery Selectors ?
What is Happening When We use Selector ?
- jQuery Traverses through all Elements of DOM every time
How Can Overcome this?
- You should Always Cache your Selections to some variable
Its Like this :
var myList = $('.myList');
myList.text("cached");
When to Use ?
- Using the Same Selection More than Once in your DOM Tree.
- e:g: Inside a jQuery Function Likes below
BAD Way
$(".myList").click( function() {
$(".myList").hide();
});
BEST Way
var myList = $(".myList");
myList.click( function() {
myList.hide(); // No need to re-select .myList since we cached it
});
More Tips for Optimizing jQuery Selector Performance :
1. Qualify your Selector strings when searching by class
- give Selector's Context as 2nd Parameter
- give Selector's Context as 2nd Parameter
- when the branches of your DOM tree become very deep
What is a Context ?
- jQuery has an optional second argument called context
- Its Limit the search within a specific node
- Great when you have a very Large DOM tree and need to find
e.g: All the <p> tags within a specific part of the DOM tree
- You can check to see what is the context of Selector by using context
Property.
e.g: $('p').context; //out put is => document
BAD Way
$(".your-class")
BEST Way
// get the node for the context
// get the node for the context
var context = $('#myContainer')[0];
$(".your-class", context )
2. Don't Qualify your selector when searching by Id.
- searching by Id uses the browsers native getElementById method (which is very fast)
BAD Way
$("div#your-id")
BEST Way
$("#your-id")
Conclusion
- Always Try to use Id of a Selector when its possible
- Always Do Caching for jQuery Selectors before it use
- Always Use Context with class Selectors
I hope this helps to You.Comments and feedback greatly appreciated.
No comments:
Post a Comment